Handling forms in Django
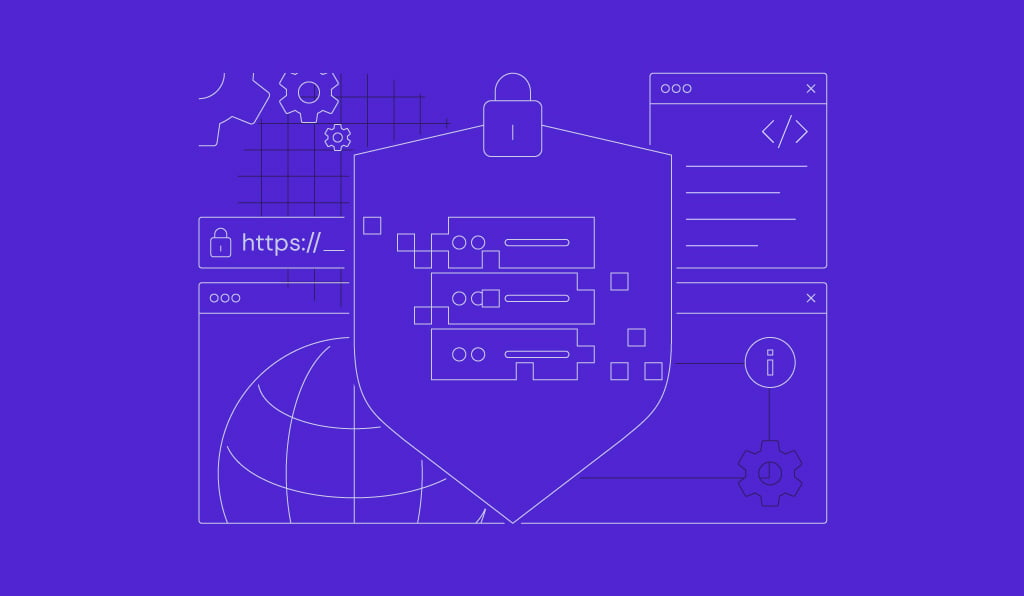
Django’s form-handling system is built on Python classes that represent forms as objects. These forms can be rendered in templates, validated, and processed using just a few lines of code.
In this article, you’ll learn how to create forms, handle form submissions, and use Django formsets.
Creating forms in Django
You can create forms either manually with Django’s Form class or automatically generate them from models using ModelForm.
To create a form in Django, define a form class in your forms.py file. This class specifies the form fields and any validation rules to process user input effectively.
Creating forms manually with forms.Form
Here’s an example of creating a simple contact form manually:
# forms.py from django import forms class ContactForm(forms.Form): name = forms.CharField(max_length=100, label='Your Name') email = forms.EmailField(label='Your Email') message = forms.CharField(widget=forms.Textarea, label='Your Message')
This form includes three fields: name, email, and message. Each field is defined using Django’s form field classes:
- CharField for short text inputs like the name.
- EmailField for email validation.
- CharField with a Textarea widget for longer text inputs, such as messages.
Generating forms from models using ModelForm
In many cases, forms are tied to models. Django simplifies this by letting you create forms directly from models using ModelForm. This saves time by automatically generating form fields based on the model’s fields.
- Creating a ModelForm form:
If you have a Post model in your models.py file:
# models.py from django.db import models class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() created_at = models.DateTimeField(auto_now_add=True)
You can create a form for this model as follows:
# forms.py from django import forms from .models import Post class PostForm(forms.ModelForm): class Meta: model = Post fields = ['title', 'content']
- Using the ModelForm form in a view:
Next, use the form in your Django view like this:
# views.py from django.shortcuts import render from .forms import PostForm def create_post(request): if request.method == 'POST': form = PostForm(request.POST) if form.is_valid(): form.save() # Saves the form data to the database return redirect('post_list') # Redirect to a list of posts else: form = PostForm() return render(request, 'create_post.html', {'form': form})
With ModelForm, Django automatically generates form fields based on the model’s fields and handles saving the form to the database with minimal boilerplate code.
Rendering forms in Django templates
To display a form on a webpage, you need to render it in a template. Django provides two approaches: using built-in rendering helpers or rendering the form manually with HTML.
Simple rendering with helper functions
Django’s built-in helper functions make it easy to customize how forms are displayed in templates. There are three methods to render a form with different structures:
- form.as_p – wraps each form field in a <p> tag, ideal for quick and clean styling.
- form.as_table – displays form fields in an HTML table, useful for structured layouts.
- form.as_ul – wraps each field in <li> tags for list-style formatting.
Here’s an example of using form.as_table:
<form method="post"> {% csrf_token %} {{ form.as_table }} <button type="submit">Submit</button> </form>
- {% csrf_token %} – adds a CSRF token to protect against cross-site request forgery attacks. This is required for forms that use POST submission.
Custom rendering with HTML
Instead of using Django’s helper methods like form.as_p, you can directly access each field using its name, such as form.name. This is ideal when you need to integrate forms into a custom-designed HTML structure or apply advanced CSS styling.
Below is an example of a full custom HTML layout:
<form method="post" action="/submit-form/"> {% csrf_token %} <div class="form-group"> <label for="id_name">Name</label> <input type="text" id="id_name" name="name" class="form-control" value="{{ form.name.value|default:'' }}"> {% for error in form.name.errors %} <div class="error">{{ error }}</div> {% endfor %} </div> <div class="form-group"> <label for="id_email">Email</label> {{ form.email }} {% for error in form.email.errors %} <div class="error">{{ error }}</div> {% endfor %} </div> <div class="form-group"> <label for="id_message">Message</label> {{ form.message }} {% for error in form.message.errors %} <div class="error">{{ error }}</div> {% endfor %} </div> <button type="submit" class="btn btn-primary">Send Message</button> </form>
You can use Django widgets to add CSS classes or attributes to form fields directly in your forms.py, for instance:
from django import forms class ContactForm(forms.Form): name = forms.CharField(widget=forms.TextInput(attrs={'class': 'form-control'})) email = forms.EmailField(widget=forms.EmailInput(attrs={'class': 'form-control'})) message = forms.CharField(widget=forms.Textarea(attrs={'class': 'form-control'}))
Then, in your template:
<form method="post"> {% csrf_token %} {{ form.name }} {{ form.email }} {{ form.message }} <button type="submit">Submit</button> </form>
Handling form submissions in Django views
Django’s forms include built-in validation to ensure submitted data is correct and secure. For example, EmailField automatically validates input to ensure it is in a valid email format.
You can also define custom validation rules to handle more specific requirements, either using field-level or form-level validation.
Validating and processing forms
Built-in validation
Django simplifies form validation by performing built-in checks automatically. Here are two key validation mechanisms:
- Required fields – by default, Django requires all form fields to have input unless explicitly set to required=False. This ensures critical fields are not left blank.
- Field types – Django validates input types based on the field definition. For example:
- EmailField checks for a valid email address.
- IntegerField ensures the input is a valid integer.
- URLField validates that the input is a properly formatted URL.
Custom validation
Django lets you define custom validation logic to enforce specific rules not covered by its built-in validation:
- Field-level validation – define a clean_<fieldname>() method for custom validation of a specific field.
- Form-level validation – override the clean() method to apply logic that considers multiple fields or general rules.
For example, to add a custom field validation for the name field:
from django import forms from django.core.exceptions import ValidationError class ContactForm(forms.Form): name = forms.CharField(max_length=100, label='Your Name') email = forms.EmailField(label='Your Email') message = forms.CharField(widget=forms.Textarea, label='Your Message') def clean_name(self): name = self.cleaned_data.get('name') if "spam" in name.lower(): raise ValidationError("Invalid name: 'spam' is not allowed.") return name
Here, the clean_name() method checks if the name field contains the word spam (case-insensitive). If it does, Django raises a ValidationError.
This error will be displayed to the user when the form is rendered again after submission.
Customizing form error display
You can customize how form errors are displayed in templates. Django automatically associates error messages with their corresponding fields, and you can manually render them in your templates for greater control over the layout like this:
<form method="post"> {% csrf_token %} <div> {{ form.name.label_tag }} {{ form.name }} {% if form.name.errors %} <div class="error">{{ form.name.errors }}</div> {% endif %} </div> <div> {{ form.email.label_tag }} {{ form.email }} {% if form.email.errors %} <div class="error">{{ form.email.errors }}</div> {% endif %} </div> <div> {{ form.message.label_tag }} {{ form.message }} {% if form.message.errors %} <div class="error">{{ form.message.errors }}</div> {% endif %} </div> <button type="submit">Send Message</button> </form>
This ensures users see relevant error messages directly next to the fields they need to correct, enhancing the form’s usability and accessibility.
Displaying form errors in templates
When working with forms in Django, it’s crucial to provide users with clear feedback on validation errors. Django simplifies this by attaching error messages to form fields, which can be displayed in templates.
Using the default error messages
Django automatically generates default error messages for invalid input based on the field type and validation rules. These messages ensure basic usability without requiring additional customization:
- This field is required. for required fields left blank.
- Enter a valid email address. for invalid input in an EmailField.
- Ensure this value has at most X characters. for exceeding the max_length limit in a CharField.
Here’s an example of displaying default error messages:
<form method="post"> {% csrf_token %} <div> {{ form.name.label_tag }} {{ form.name }} {{ form.name.errors }} </div> <div> {{ form.email.label_tag }} {{ form.email }} {{ form.email.errors }} </div> <div> {{ form.message.label_tag }} {{ form.message }} {{ form.message.errors }} </div> <button type="submit">Send Message</button> </form>
Customizing error messages
While Django’s default messages are useful, you might want to make them more specific or tailored to your audience. You can do this by setting the error_messages parameter when defining fields in your form class.
For instance, to set up field-level custom error messages in forms.py:
from django import forms class ContactForm(forms.Form): name = forms.CharField( max_length=100, error_messages={ 'required': 'Please enter your name.', 'max_length': 'Name must not exceed 100 characters.' } ) email = forms.EmailField( error_messages={ 'required': 'We need your email address to contact you.', 'invalid': 'Please enter a valid email address.' } ) message = forms.CharField( widget=forms.Textarea, error_messages={'required': 'Don’t forget to include a message!'} )
Using Django formsets
A formset is a layer of abstraction over multiple forms, making it easier to create, validate, and process several forms simultaneously. With formsets, you can efficiently manage multiple instances of a form on a single page.
Formsets are particularly useful for handling related data or dynamic forms, such as adding multiple entries to a database at once. Django simplifies this process with the formset_factory utility function, which generates a formset class from a single form class.
Creating a formset
To create a formset, define a base form in the forms.py file:
# forms.py from django import forms class ItemForm(forms.Form): name = forms.CharField(max_length=100) quantity = forms.IntegerField(min_value=1)
Then, generate a formset using formset_factory in views.py:
# views.py from django.shortcuts import render from django.forms import formset_factory from .forms import ItemForm def manage_items(request): ItemFormSet = formset_factory(ItemForm, extra=3) # Create 3 empty forms by default if request.method == "POST": formset = ItemFormSet(request.POST) if formset.is_valid(): for form in formset: # Process each form's cleaned data print(form.cleaned_data) return redirect('success') # Redirect after processing else: formset = ItemFormSet() return render(request, 'manage_items.html', {'formset': formset})
Using model formsets
If the formset is tied to a model, you can use Django’s modelformset_factory to generate a formset directly from a model class, for example:
from django.forms import modelformset_factory from .models import Item def manage_items(request): ItemFormSet = modelformset_factory(Item, fields=('name', 'quantity'), extra=3) if request.method == "POST": formset = ItemFormSet(request.POST) if formset.is_valid(): formset.save() # Save all forms at once return redirect('success') else: formset = ItemFormSet() return render(request, 'manage_items.html', {'formset': formset})
Conclusion
Django forms simplify handling user input with built-in validation, customizable error messages, and rendering flexibility. In this guide, we’ve demonstrated how to create forms manually, generate them from models, and manage multiple forms with formsets.
You can also leverage advanced features like formset_factory, custom validation methods, and model formsets to create user-friendly, robust forms for complex data handling scenarios. If you still have questions about handling Django forms, feel free to use the comment box below!
Django forms FAQ
What are Django forms?
Django forms are classes that simplify handling user input by generating HTML form elements, validating data, and processing submissions. They support custom fields, built-in validation, and error handling. Django forms streamline data collection and integrate input directly with models for efficient data management.
How do I create a form in Django?
To create a form in Django, define a class that inherits from forms.Form or forms.ModelForm in your forms.py file. Add fields as class attributes, such as CharField or EmailField. For model-based forms, ModelForm automatically maps fields to the specified model, simplifying data handling.
How can I validate form data in Django?
Django automatically validates form data using built-in validators when is_valid() is called on a form instance. Add custom validation with clean_<fieldname> methods for specific fields or clean() for the entire form. This ensures data meets requirements before saving or processing further.